Platforms
Turning big ideas for tomorrow's businesses.
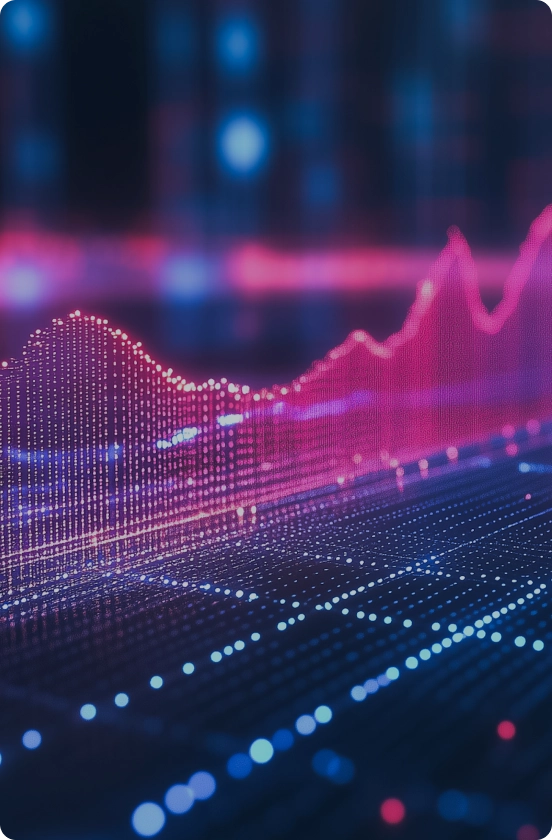
FinTech
Secure, seamless digital payments in 45+ countries.
mobiquity® Pay
explore fintech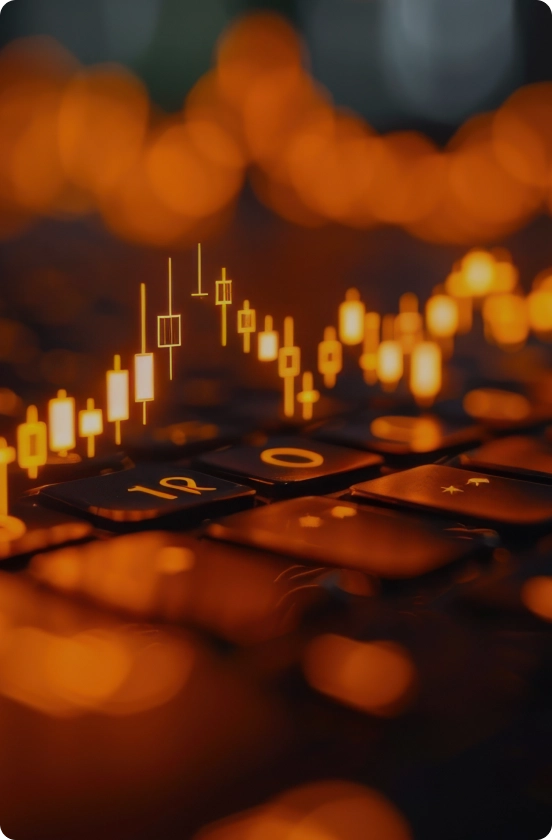
MarTech
AI-driven marketing automation for hyper-personalized experiences.
MobiLytix™ Digital
discover Martech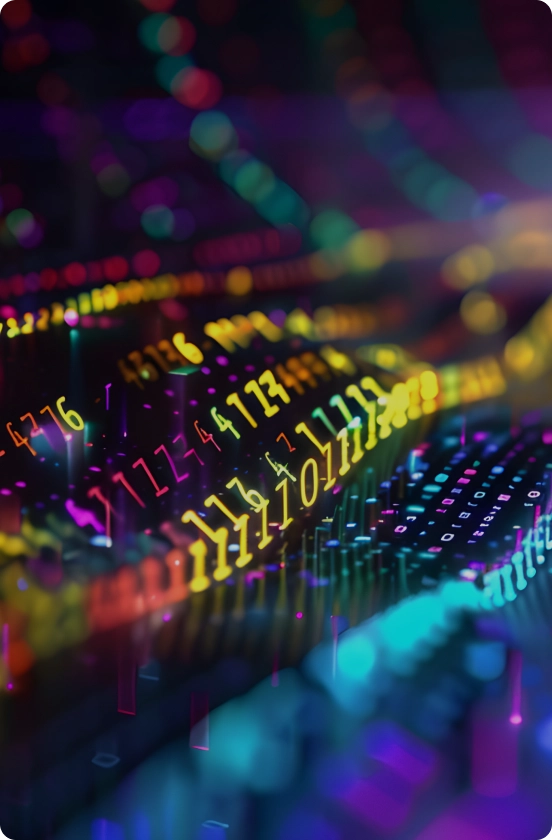
DigiTech
Simplify growth with intelligent business platforms tailored for telecom and beyond.
BlueMarble BSS
explore Digitech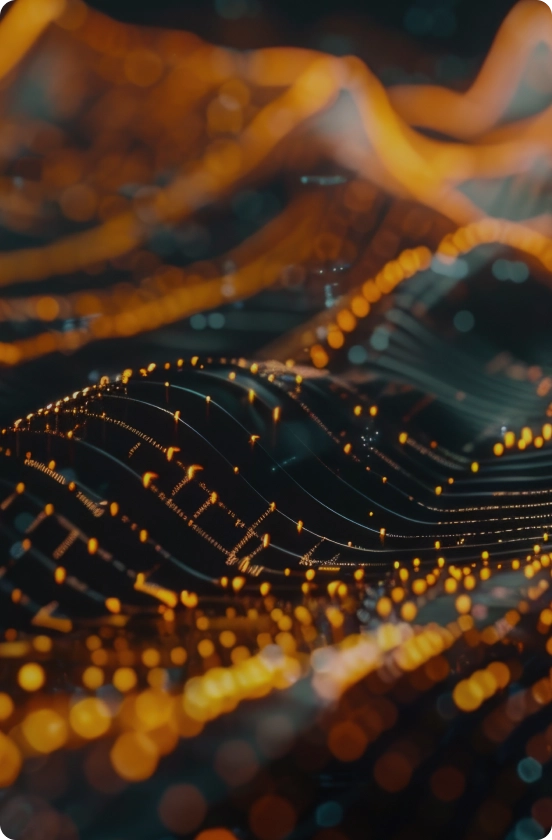
RevTech
Gen-AI-powered platform unlocking telco potential.
CNPaaS
explore revtechCredible Impact
Driving global success that delivers lasting impact.
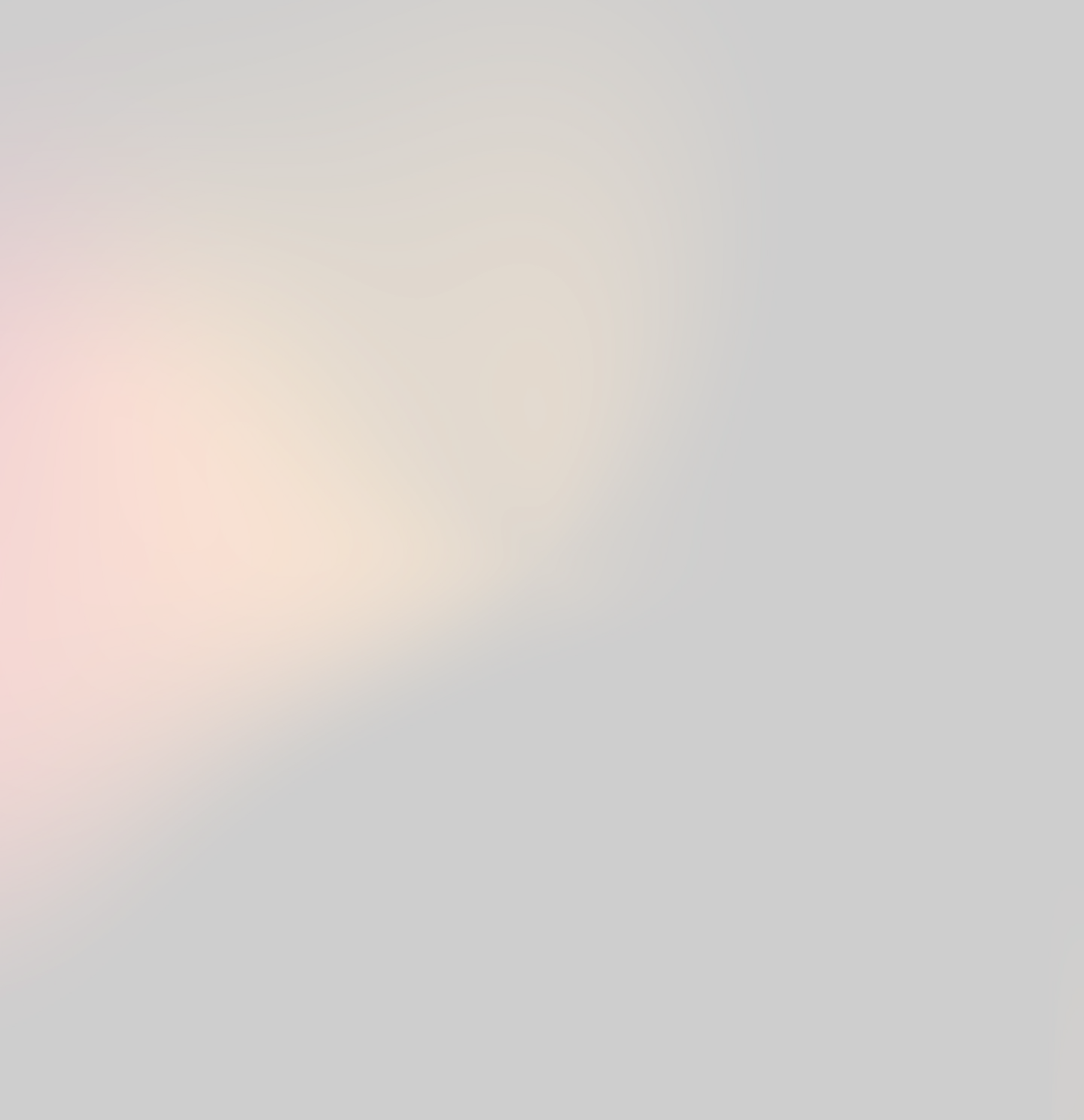
Trusted by Industry Leaders
Recognized for our work
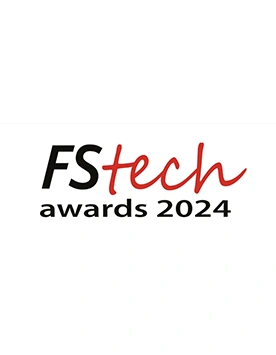
FStech Award 2024
Financial Inclusion
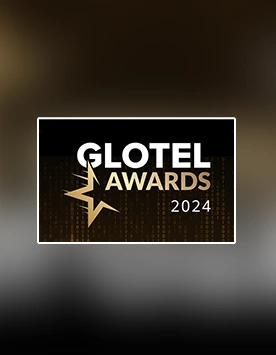
GLOTEL Award
Harnessing Network Exposure
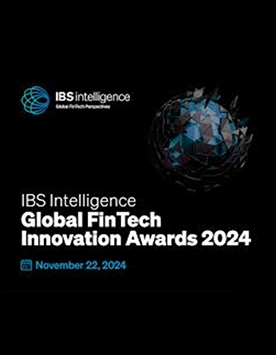
IBSi Global FinTech Innovation Award
Most Innovative Digital Wallets Deployment for our work with Dahabshiil Inc
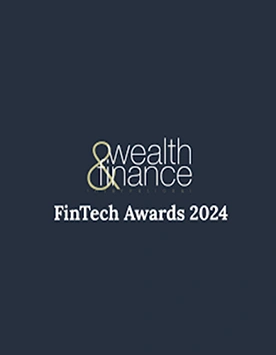
Wealth and Finance International
Digital Money & Payment Platform of the Year 2024 – India
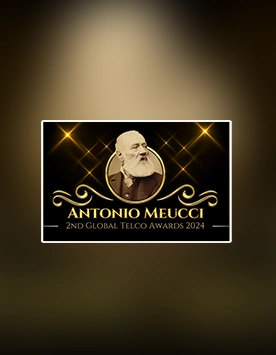
Antonio Meucci Award
Best Cpaas Provider
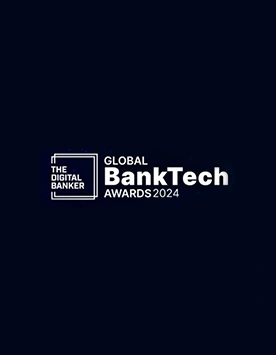
Global BankTech Award
Best Retail Banking Solutions Provider
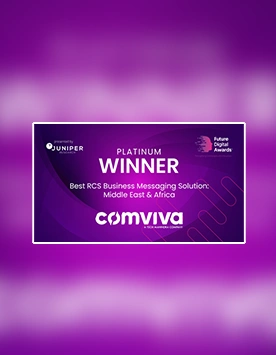
Juniper Research Telco Innovation Award
Best RCS Business Messaging Solution ME & Africa
A legacy of innovation, a world of impact
At Comviva, our software platforms are shaping the digital landscape of business growth and customer experience across the globe.
About Comviva25+
Years of technological excellence
2Bn+
Platform users across the world
100+
Countries served
3000+
Thinkers, Researchers & Innovators